Serialize And Deserialize A Given N-Ary Tree
Given key and NULL children pointer. Void serialize(Node *root, FILE *fp). // Else, store. Design an algorithm to serialize and deserialize a binary tree. There is no restriction on how your serialization/deserialization algorithm should work.
- // A C++ Program serialize and deserialize an N-ary tree
- #define MARKER ')'
- usingnamespace std;
- // A node of N-ary tree
- char key;
- Node *child[N];// An array of pointers for N children
- // A utility function to create a new N-ary tree node
- {
- temp->key = key;
- temp->child[i]=NULL;
- }
- // This function stores the given N-ary tree in a file pointed by fp
- {
- if(root NULL)return;
- // Else, store current node and recur for its children
- for(int i =0; i < N && root->child[i]; i++)
- fprintf(fp, '%c ', MARKER);
- // This function constructs N-ary tree from a file pointed by 'fp'.
- // This functionr returns 0 to indicate that the next item is a valid
- int deSerialize(Node *&root, FILE*fp)
- // Read next item from file. If theere are no more items or next
- char val;
- return1;
- // Else create node with this item and recur for children
- for(int i =0; i < N; i++)
- break;
- // Finally return 0 for successful finish
- }
- // A utility function to create a dummy tree shown in above diagram
- {
- root->child[0]= newNode('B');
- root->child[2]= newNode('D');
- root->child[0]->child[1]= newNode('F');
- root->child[2]->child[1]= newNode('H');
- root->child[2]->child[3]= newNode('J');
- root->child[0]->child[1]->child[0]= newNode('K');
- }
- // A utlity function to traverse the constructed N-ary tree
- {
- {
- for(int i =0; i < N; i++)
- }
- int main()
- // Let us create an N-ary tree shown in above diagram
- // Let us open a file and serialize the tree into the file
- if(fp NULL)
- puts('Could not open file');
- }
- fclose(fp);
- // Let us deserialize the storeed tree into root1
- fp =fopen('tree.txt', 'r');
- traverse(root1);
- return0;
Embed

// |
// 40 |
// 20 21 22 |
//10 11 12 13 14 |
// 2 |
//serialised value is : 40 20 10 ) 11 2 ) ) ) 21 ) 22 12 ) 13 ) 14 ) ) ) |
// A C++ program to demonstrate serialization and deserialiation of |
// n-ary Tree |
#include<iostream> |
#include<vector> |
usingnamespacestd; |
//char MARKER=')'; |
#defineMARKER -1 |
/* A binary Node has key, vector of Node * for children */ |
structNode |
{ |
int key; |
vector< structNode * > child; |
}; |
/* Helper function that allocates a new Node with the |
given key and NULL children pointer. */ |
Node* newNode(int key) |
{ |
Node* temp = new Node; |
temp->key = key; |
return (temp); |
} |
// This function stores a tree in a file pointed by fp |
voidserialize(Node *root, FILE *fp) |
{ |
// Else, store current node and recur for its children |
fprintf(fp, '%d ', root->key); |
unsigned i=0; |
while(i<root->child.size()) |
{ |
serialize(root->child[i], fp); |
i++; |
} |
fprintf(fp, '%d ', MARKER ); |
} |
// This function constructs a tree from a file pointed by 'fp' |
// 40 20 10 ) 11 2 ) ) ) 21 ) 22 12 ) 13 ) 14 ) ) ) |
voiddeSerialize(Node *&root, FILE *fp) |
{ |
} |
/* |
// A simple inorder traversal used for testing the constructed tree |
void inorder(Node *root) |
{ |
if (root) |
{ |
inorder(root->left); |
printf('%d ', root->key); |
inorder(root->right); |
} |
} |
*/ |
voidprintTree(structNode * root) |
{ cout<< root->key<<''; |
unsigned i=0;//becoz vectoe size returns unsigned int |
while(i < (root->child).size()) |
{ |
printTree(root->child[i]); |
i++; |
} |
cout<<endl; |
} |
/* Driver program to test above functions*/ |
intmain() |
{ |
// Let us construct a tree shown in the above figure |
structNode *root = newNode(40); |
root->child.push_back( newNode(20) ); |
root->child.push_back( newNode(21) ); |
root->child.push_back( newNode(22) ); |
root->child[0]->child.push_back(newNode(10)); |
root->child[0]->child.push_back(newNode(11)); |
root->child[0]->child[1]->child.push_back(newNode(2)); |
root->child[2]->child.push_back(newNode(12)); |
root->child[2]->child.push_back(newNode(13)); |
root->child[2]->child.push_back(newNode(14)); |
//level order traversal of tree |
printTree(root); |
// Let us open a file and serialize the tree into the file |
FILE *fp = fopen('tree.txt', 'w'); |
if (fp NULL) |
{ |
puts('Could not open file'); |
return0; |
} |
serialize(root, fp); |
fclose(fp); |
// Let us deserialize the storeed tree into root1 |
Node *root1 = NULL; |
fp = fopen('tree.txt', 'r'); |
deSerialize(root1, fp); |
/*printf('Inorder Traversal of the tree constructed from file:n'); |
inorder(root1);*/ |
return0; |
} |
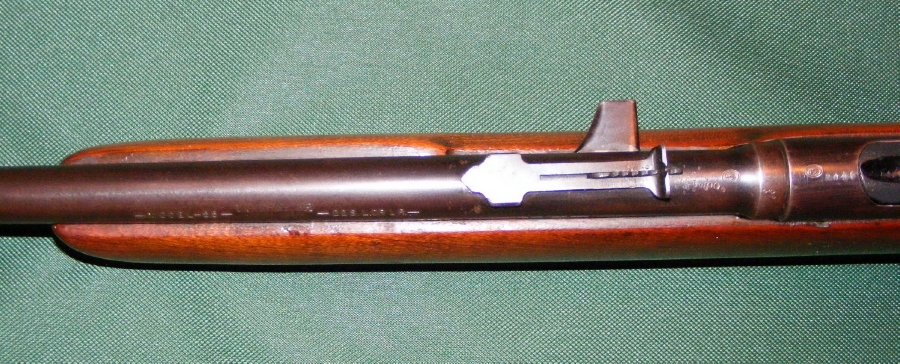
commented Nov 15, 2014
De serialize part is remaining to implement |
commented Nov 15, 2014
commented Nov 18, 2014
int deSerialize(Node *&root, FILE *fp) } The System Timer, however, is supplied to the priority encoder through a latch that is reset by software. 3b2 tutorial pdf. Table 35: Full-interrupt vector table in ROM Address Contents IPL Notes 0x8C 0x02000BC8 NMI Handler 0x90 0x02000BC8 0 Auto-Vector Handler (not used) 0x94 0x02000BC8 1 PCBPs (31 words) 0xAC 0x02000C18 8 Programmed Interrupt 8 (PIR8) 0xB0 0x02000C68 9 Programmed Interrupt 9 (PIR9) 0xB4 0x02000CB8 0xB8 0x02000D08 11 Hard Disk & Floppy 0xBC 0x02000D58 0xC0 0x02000DA8 13 UART 0xC4 0x02000DA8 0xC8 0x02000E48 15 10ms interval timer and Syserr 0xCC 0x02000BC8 0xD0 0x02000BC8 0x10C 0x02000BC8 Device Interrupt Handler 0x110 0x02000BC8 PCBPs (224 words) (All identical PCBPs). The 3B2 interrupt subsystem takes interrupt inputs from several sources on the system board and translates them into values on the CPU's four bit IPL (Interrupt Priority Level) bus. The IPL bus is fed by a 74LS148 priority encoder. The hard disk controller (ID), floppy disk controller (IF), and DUART (IU) interrupt outputs are directly connected to the priority encoder (potentially through inverters), and are cleared when the originating device de-asserts its IRQ line. |